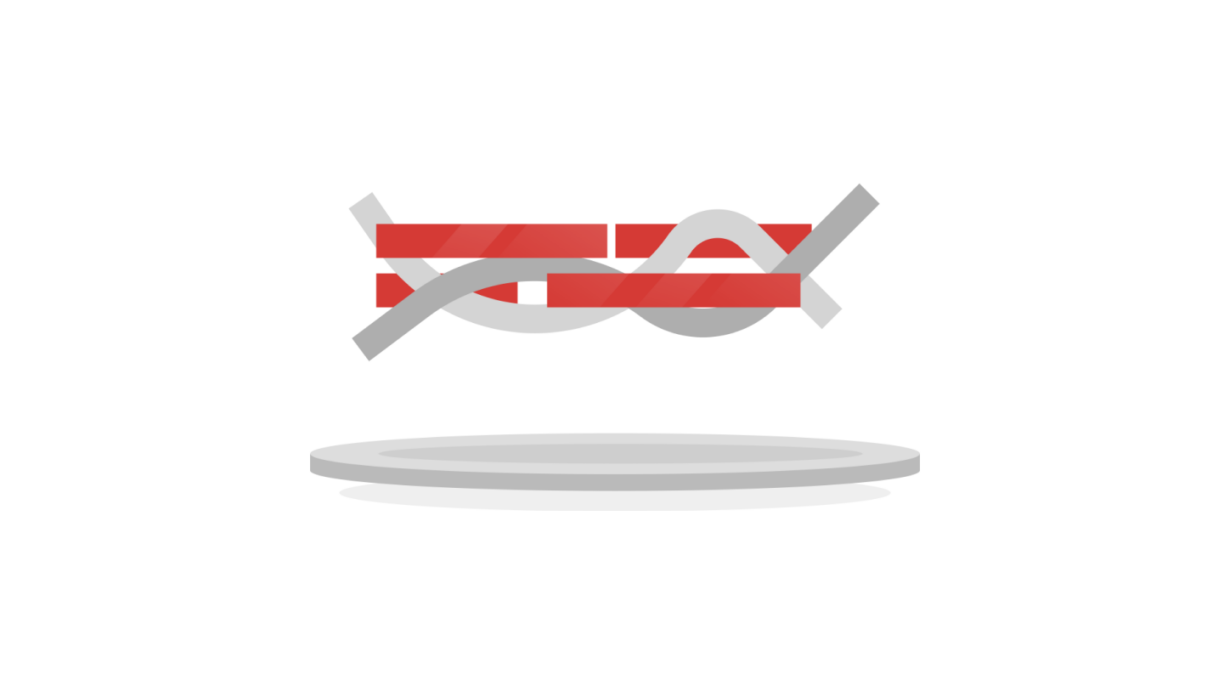
Async/await – how it works
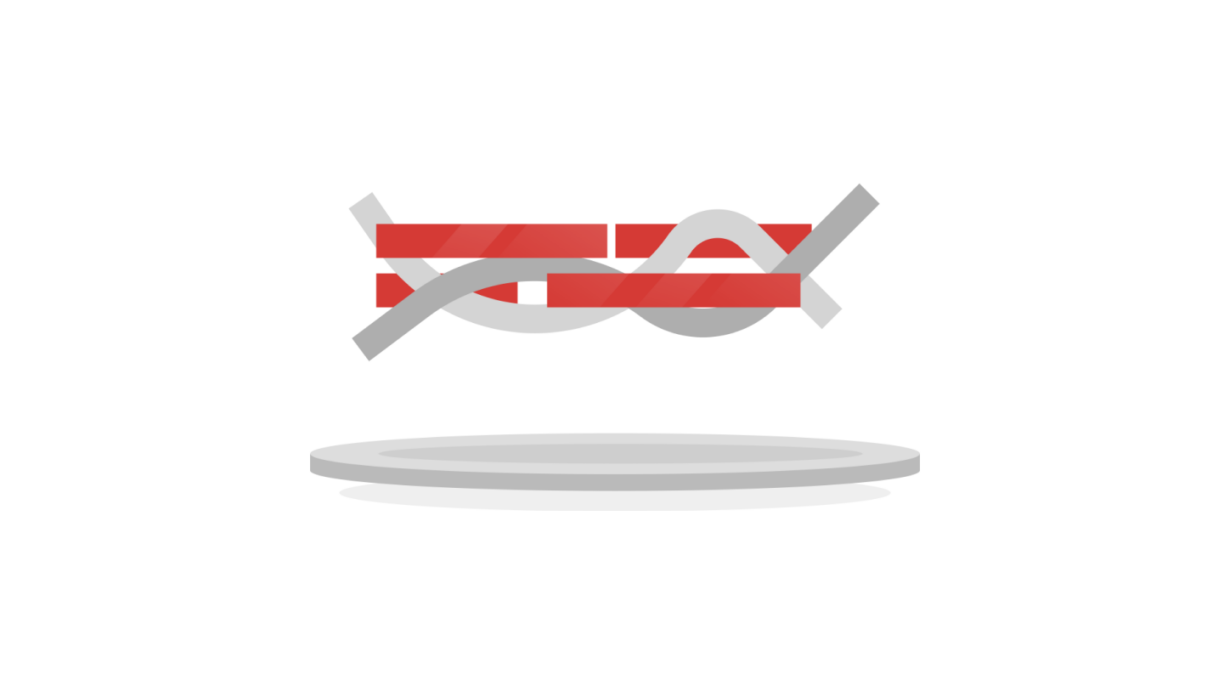
Async/await is a brand new way of handling asynchronous calls in JavaScript. If you don’t know how it works, in this article I will be providing some examples to help you get started.
Async/await – how it works
JavaScript async/await is a relatively new way to handle asynchronous operations in JavaScript. It gives you power
new to make your code shorter and more understandable.
Before async/await, callbacks and promises were used to handle asynchronous calls in JavaScript. Everyone who learns JavaScript has heard about the so-called callback hell. However, I won’t be writing about this because callbacks aren’t the main focus of this post.
Promises for beginners
As you can see in the example above, I need to make a requestMock
function on the stub to call an asynchronous operation. It’s a perfect example of using setTimeout
to make a long-running process asynchronous operation.
The promise object – which is returned fromrequestMock
– uses the then
method. This method gives you the possibility to handle the asynchronous execution, in the case that requestMock
ends successfully.
It’s worth mentioning that the promise uses not only thethen
method but also the catch
method. Thecatch
the method enables you to handle asynchronous operations that end unsuccessfully.
You will need to use requestMock()
with the `then` method to handle with your asynchronous operations.
Rewrite a promise to Async/Await
As you can see, in the example above I have used the async
keyword which means that this function is asynchronous and returns the promise. Whenever you use async/await, make sure you’re using the try/catch statement to handle errors.
You should also use await
to wait for the end of requestMock
and declare res
to save the returned value. ‘Await’ must be called inside async
. Withoutawait
, the asynchronous function will end and it will not wait for the result of the asynchronous function.
Key points to keep in mind
- Both async/await and the promise play the same role, but in the first case, use the `then` syntax, whereas in the second case use the async/await syntax.
- Async/await is more readable and more comfortable to write.
- Async/await is – like promise – non-blocking.
- When you decide to use async/await, it’s good practice to use
tryCatch
to handle errors. With promise, you have to use onlycatch
to handle an error. - Be careful! It’s easy to get lost with a promise if you have a lot of nesting (6 levels) bracers and the statements are returned. With async/await you won’t have this problem.
When you declare async
, you can use the await
keyword to make your code in a synchronous fashion. The code will look very similar to its synchronous counterpart, but it will still be asynchronous.
However, the important fact is that you will still need promises to write asynchronous code.
Async/await with promises
As I have already mentioned, an async
function returns a promise. So you can write something like this:
Therefore, you can combine the promise.all
and async/await to make a lot of many requests:
Let’s imagine that you have to limit asynchronous operations in time. I usually use the async library to do it. One of the functions in this library is mapLimit.
MapLimit gives you the opportunity to carry out asynchronous operations if you want to do some operations at the same time but not all of them at once.
In the example above, you can see a standard request
function to stub the long background process. At this stage, you can create the mapLimit
function. In the mapLimit function, you can slice up an array of functions into a chunk array. Subsequently, you can use the for-of loop which was added in ES6 to access the elements of the array.
In the loop, it’s helpful to use await Promise.all
because you want to process the requests concurrently. This is possible because, at the beginning of the function, the main array is divided into arrays of chunk functions.
Congratulations! You now have the generic mapLimit function. You can use it every time you have to process anything or something concurrently!
How to implement async/await with streams?
Node.js 10 was released on April 24, 2018. The publication of Node.js 10 was a kind of breakthrough because this version allows you to use async/await
, when you operate on streams.
It’s also worth mentioning that the ES2018 version allows the possibility to carry out an asynchronous iteration.
Usually, your streams look like this:
Node.js 10 gives you the possibility to use an asynchronous iteration to read a file asynchronously. You can iterate streams only if you declare async
ahead of the function. More examples can be found on this page: 2ality.com
You see? Everything is understandable and readable. In this example, I used the for-await-of loop to iterate. This operation is possible thanks to the Symbol.asyncIterator
To start, let’s take a look at Symbol.iterator
`Async Iterators `are similar to regular iterators in that they implement 3 methods: the next method moves to the next item (the rest of the bullet list) and eventually a return or a throw method.
There are some differences between an `async iterator` and a conventional `iterator`. Instead of returning a plain object { value, done }
, an async iterator returns a promise which is the counterpart of { value, done }
.
Similarly, an async iterable is an object with a Symbol.asyncIterator
function that returns an asynchronous iterator. It enables you to change the syntax of `for…of` into an asynchronous for await...of
These are already implemented in recent versions of Node.js, and you can apply them by using the --harmony-async-iteration
flag.
Conclusion
You can write code both in a synchronous fashion and intuitively way.
async/await
is a syntax that was added in ES8 (ECMAScript 2017) of the JavaScript version. It’s supported by all modern browsers. Node.js programmers can use this syntax from the Node.js 8 version.
As you can see, the async/await
the syntax is not at all complicated. It’s just another way of working with the promise object, and thanks to this you’re now able to write code much more synchronously and intuitively fashion.
It’s also important to remember that the ‘Async’ function returns a promise but ‘await’ can only be used inside the async block.
See also