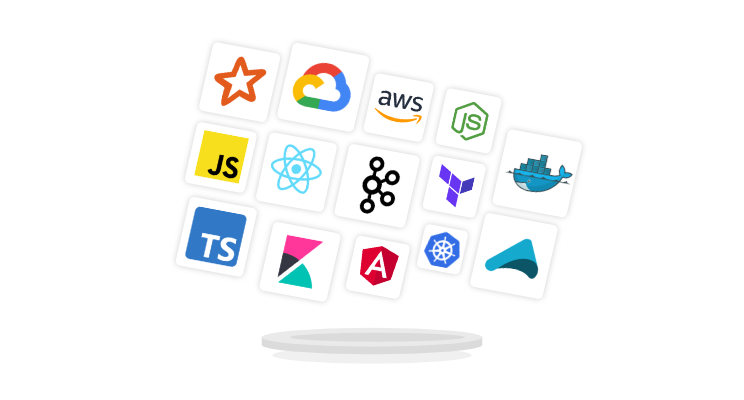
Using Reactive Extensions for data binding in Scala.js
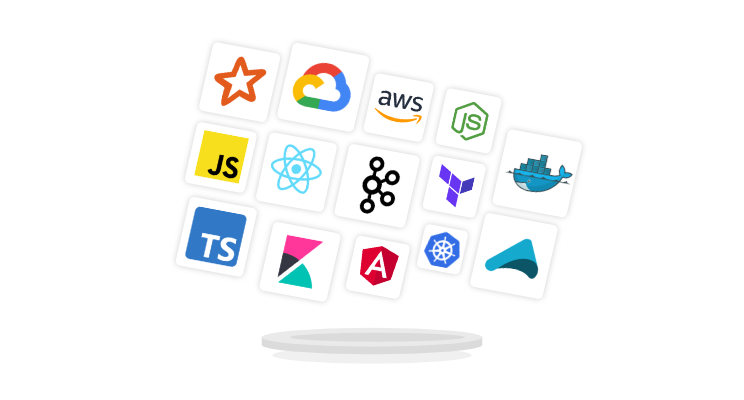
The Problem
In my previous post we have written a simple app with Scala.js. In the end we managed to do everything we wanted to, but as we added more and more code a problem appeared – state management, the real app killer. Although our app didn’t do anything advanced, state handling and UI updates forced us to write a lot of additional code.
That post has shown that Scala.js allows us to write browser apps, but it also demonstrated that these apps can be vulnerable to other ills of frontend programming. To fix this issue we need to simplify our codebase. One way to do that is by adding a binding between the UI and what data our app holds. This way if the data changes in one place it will be automatically propagated to the other. We will implement this using Reactive Extensions.
What are Reactive Extensions?
Reactive Extensions (Rx for short) is a library originally introduced by Erik Meijer to the .NET framework. Rx aims to allow the composition of asynchronous and event-based programs using Observables. It might sound confusing at first, but the idea gets intuitive after you try it out. Observable can be seen as a source of events, hence “event-based”.
We don’t know when an event might happen, that’s why it’s “asynchronous”. We can observe the source and react on events, thus sources are called Observable.
Consider an app that counts the number of clicks on a button. The button will be our Observable and it will emit a “Click” event each time it gets clicked. Using Rx we can create a stream of “Click” events where each will update a counter. This is the idea behind Reactive Extensions in a nutshell.
After being introduced to .NET the idea quickly spread across other languages, including Scala. In our post we will use Scala.Rx created by Li Haoyi. We’ve chosen this implementation as it’s fully compatible with Scala.js. It’s no surprise if you consider that creator of Scala.Rx is also the person behind Scala.js.
Reactive Implementation
To simplify our organizer we want to create a graph that will propagate changes in our data from one side of the app to another. We will aim to create a graph as depicted below.
Round shapes represent event sources of various kinds – time, user input, and web. Even our TODO data might be used as an event source. Events are then passed to calculations – block shapes with round edges. Based on the calculated values we update the UI. I will explain it step by step. Let’s start from our sources. In Scala.Rx we create them using Var
s as follows:
https://gist.github.com/pjazdzewski1990/39c1c302974aac08012e
It get’s slightly more complicated with other Var
s, so let’s define a helper trait.
https://gist.github.com/pjazdzewski1990/2b87b85eaf411301e172
That looks quite complicated at first glance, but really it isn’t. All we do here is create a Var
that updates when a browser event happens – interval tick or elements onClick
handler. We will put those helpers to good use in our main code.
https://gist.github.com/pjazdzewski1990/3c3b317e1877b0fa84e5
Having that in place, we can add dependent calculations. In order to make Scala.Rx do it’s magic we need to put all calculations depending on sources inside Rx
blocks or Obs
. It’s as simple as:
https://gist.github.com/pjazdzewski1990/a23d3988759aa8b32ca7
If you compare this new solution with the diagram above, you will find out that they are basically the same. Thanks to Rx we’ve turned a stateful spaghetti code into a simple flow. Neat.
What is the difference between
Rx
andObs
?
Rx
is a definition that automatically capturesVar
s and otherRx
s in it’s block. When those captured elements change, the capturing block is evaluated again with the new values and the capturingRx
is updated.Obs
can be thought as a more advancedRx
. You can do a few more tricks with it – like perform side effects and explicitly define dependencies (which we use in this example).
Summary
As you can see Rx was immensely useful for reducing boilerplate code and creating a simple flow of values in the system. Reactive Extensions have proven their worth.
Creating this bidirectional flow is a step in the right direction – it helped us to manage state. Still when the app grows so does the graph and potentially it may get big and complicated later on. Modern frontend apps deal with this issue by using encapsulated components and unidirectional flows … but it’s something for another post.
PS. Special thanks to lihaoyi for creating the great code – Scala.Rx. It wouldn’t be possible without you.
[UPDATE] I would like to say sorry to Sébastien Doeraene who is the author of Scala.js and was omitted in these blog posts. Without his hard work Scala.js would not come to life. Thank you Sébastien.
Links
- https://lihaoyi.github.io/hands-on-scala-js/#Functional-ReactiveUIs
- https://github.com/lihaoyi/scala.rx
Do you like this post? Want to stay updated? Follow us on Twitter or subscribe to our Feed.
Read more