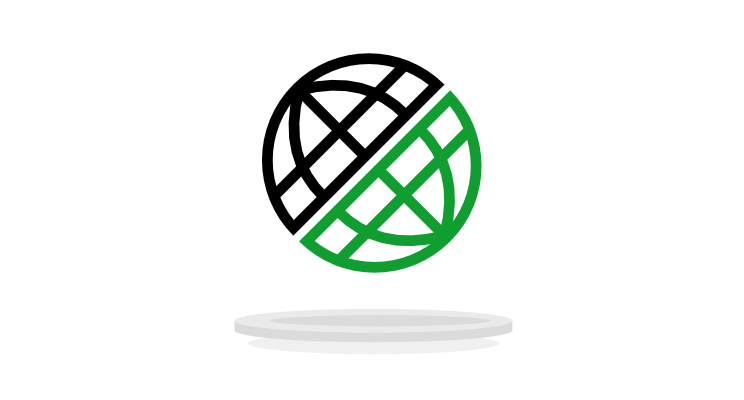
Rest Assured API Testing Tutorial. How To Use Rest Assured With Java And Scala.
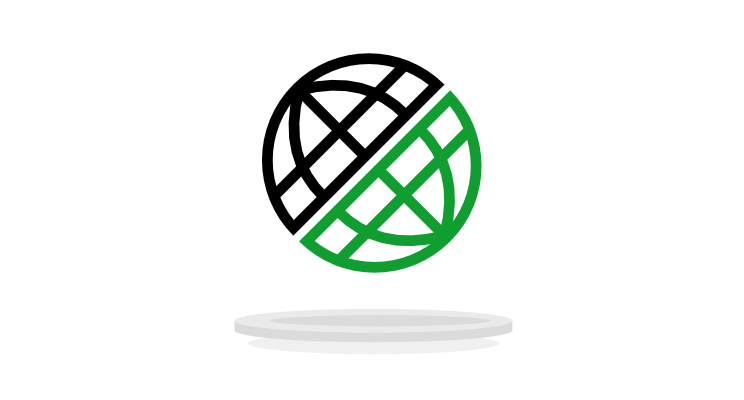
Why do you need this Rest Assured API testing tutorial? In the world of testing – automation can be a real game-changer. That’s why it’s on everyone’s tongues, especially “automated tests” in the context of GUI tests written in python/java with SeleniumWebDriver. Or possibly with the increasingly popular Cypress.io. However, GUI tests sit right at the top of the basic pyramid when it comes to testing.
The real basis lay in the unit tests written by programmers and they are also the basis when it comes to the quality of software. On the other hand, testers most often have to test the API, which doesn’t have to necessarily be automated, but may also be done through manual backend testing.
In this article, you will learn
- What is an API?
- Concept of REST vs SOAP
- What is REST Assured?
- To use or not to use?
- Rest Assured in Java
- Rest Assured in Scala
Let’s start at the beginning.
What is an API?
In simple words, API (Application Programming Interface) is a software intermediary that allows two applications to talk to each other. For example – think about websites which present from cheap airlines (like Skyscanner, for example). When we enter a phrase into the search box, the search engine does not connect directly to each airline’s database. Rather than that, those companies expose their public APIs. Those APIs allow other developers to fetch necessary data in a natural way. Without the need to understand each particular database schema.
Here are some examples of famous open APIs:
1.Google Maps API: the Google Maps API allows developers to embed Google Maps on webpages using a JavaScript or Flash interface.
Examples of apps and companies using Google Maps API:
Glovo, Snapchat, Groupon, Mastercard, Snapchat
2. YouTube APIs: YouTube APIs allow developers to integrate YouTube videos and functionality into websites or applications. YouTube APIs include the YouTube Analytics API, YouTube Data API, YouTube Live Streaming API, YouTube Player APIs, and others.
Examples of apps and companies using YouTube API:
Google App Engine, Google Analytics
3. Spotify API: the Spotify API allows developers to use audio analysis, audio playback, and more.
Examples of apps and companies using the Spotify API:
Optimizely, Blossom
Source: https://stackshare.io/feed
Concept of REST vs SOAP
After becoming familiar with the definition of API, we can smoothly switch to Internet service design models. And here I will focus on the two dominant ones, namely REST (Representational State Transfer) and SOAP (Simple Object Access Protocol). The second, SOAP, does well mainly in financial structures because it is most valued for its security and standardization as well as its simple control of data content. As for REST – or rather the REST API – some time ago this was said to be on a par with microservices and NoSQL.
REST itself as an API design standard is not a framework, but rather a set of principles (or good practices), which means that this solution is very flexible. REST is able to handle many data formats such as XML, JSON, and even YAML while SOAP can function only in XML format. These good practices were defined by Roy Fielding in his doctoral dissertation in 2000, and they look like this:
- Client-server architecture
- Statelessness -> i.e., not storing information about what happened before, you could also call it independence of connections
- Cacheability -> should have the ability to buffer client-server data online
- Layered system -> the design is divided into layers, where each of them performs a separate function
- Code on demand (optional, not yet very popular) -> allows you to send new code fragments via API to server
- Uniform interface -> separation of implementation on the client and server-side by using a uniform interface
Okay, now we know the idea of the above design models, but there is also one more important thing to underscore. Namely, when we talk about web API we need to also remember HTTP protocol and the most usable method corresponding to the CRUD (Create, Read, Update, Delete) action. The corresponding method we have here is: POST, GET, PUT, DELETE. An example of such a method might look like this:
GET: url/movies
POST: url/movies
PUT: url/movies/id
DELETE: url/movies/id
What is REST Assured?
Which brings us nicely to our main topic. Namely, one of the most popular libraries which allows us to easily automate our REST API. Rest Assured is a Java-based library that we can use to test RESTful Web Services. The fact that this is a Java-based library of course does not matter because REST Assured is based only on JSON and HTTP. These are language-independent, so with the help of REST Assured we can create tests for applications written in any language. This library behaves like a headless Client to access REST web services. We can create an extensive HTTP request in just one line. Thanks to the many options available, we can test various core business combinations.
REST Assured also provides us with a lot of validations to our requests, such as for example Status Code, Status Response, Headers, or even Body response. This means that the library is really flexible when it comes to testing.
Rest Assured uses gherkin syntax. So a request looks like this (in Java):
private String username = "username";
private String password = "password";
private String url = "https://localhost:8080/";
private String endpoint = "endpoint"
@Test
public void GetTest() {
Response response = when()
.get(url + endpoint);
}
Looks simple? For the example above we get to our endpoint, but the code lacks assertion! So let’s add some assertion, for example, for status code, so it will look like this:
private String username = "username";
private String password = "password";
private String url = "https://localhost:8080/";
private String endpoint = "endpoint";
@Test
public void GetTest() {
Response response = when()
.get(url + endpoint)
.then()
.assertThat()
.statusCode(200);
}
If someone wants to add an assertion with the help of JUnit, this is also possible:
Assertions.assertEquals(200, response.statusCode());
We can also add authorization to our code, here we have username and password:
private String username = "username";
private String password = "password";
private String url = "https://localhost:8080/";
private String endpoint = "endpoint";
@Test
public void GetTest() {
Response response = given()
.port(80)
.auth()
.oauth(username, password, "", "")
.when()
.get(url + endpoint)
.then()
.assertThat()
.statusCode(200);
}
Except for basic authorization, we can also access our API with the help of an access token, like this
private String username = "username";
private String password = "password";
private String url = "https://localhost:8080/";
private String endpoint = "endpoint";
@Test
public void GetTest() {
Response response = given()
.port(80)
.auth()
.oauth2(“abc”)
.when()
.get(url + endpoint)
.then()
.assertThat()
.statusCode(200);
}
API testing using rest assured – Hot or not?
Because of its ease of use, needing just a little bit of learning (especially for people who know Java) REST Assured is very popular. Is this solution perfect? I can’t exactly say that, but I can discuss the question in broader terms.
It is, of course, mainly recommended because it has built-in checking right away. But it also boils down to the fact that the tests are very low-level and work with JSON instead of model objects.
In the case of model objects, we receive JSON from the application being tested, transform it into model objects, and perform all of the checks based on model objects.
However, you can make a request and receive responses in JSON format, which will then later be transformed into model objects to perform REST Assured queries. The code comes out more compact than using the HTTP client, as you saw in the example above.
Rest Assured Java Tutorial
To start writing tests in REST Assured we need of course an API but also a few libraries. In the dependencies, Gradle or Maven syntax will be different, but to begin writing our tests, we will need the following (it’s recommended to download the newer more stable versions) :
- Basic https://mvnrepository.com/artifact/io.rest-assured/rest-assured
- Gson (this can convert Java Objects into their JSON representation. We can also use it to convert a JSON string to an equivalent Java object) https://mvnrepository.com/artifact/com.google.code.gson/gson
- Jackson Core (parser and generator abstractions used by Jackson Data Processor) https://mvnrepository.com/artifact/com.fasterxml.jackson.core/jackson-databind
- Scribe Java (Simple OAuth library for Java
https://mvnrepository.com/artifact/com.github.scribejava/scribejava-httpclient-okhttp
https://mvnrepository.com/artifact/com.github.scribejava/scribejava-apis
- JUnit (unit tests, here needed for the assertion)
https://mvnrepository.com/artifact/junit/junit
https://mvnrepository.com/artifact/org.junit.jupiter/junit-jupiter-api
The API that I will use in my article will be A Giter8 template for a basic Scala application build using ZIO, Akka HTTP, and Slick. This template integrates ZIO with Akka HTTP and Slick so that you can seamlessly use all power of ZIO in a familiar environment of battle-tested Akka-Http routes and slick query DSL. It also leverages ZLayer and ZManaged to build a dependency graph in a very visible, type-safe, and resource-safe way. In this API we have the ability to use two HTTP methods, GET and POST.
First of all, I created two Strings with URL and endpoint. Next, I created a request body (HashMap). “jsonAsMap” object is automatically converted into JSON with two fields: “name” and “price”. Then, I can show what GET and our POST will look like with the sent body. The last step is creating an assertion. For this example, I have used an assertion from Hamcrest and JUnit. In this example, I have also put a print line statement to the console to show a response.
import io.restassured.response.Response;
import org.hamcrest.MatcherAssert;
import org.junit.jupiter.api.Assertions;
import org.junit.jupiter.api.Test;
import java.util.HashMap;
import java.util.Map;
import static io.restassured.RestAssured.given;
import static org.hamcrest.CoreMatchers.equalTo;
public class RestTest {
private String url = "https://localhost:8080/";
private String endpoint = "items";
@Test
public void crudTest() {
Map<String, Object> jsonAsMap = new HashMap<>();
jsonAsMap.put("name", "Test12");
jsonAsMap.put("price", 50);
Response response = given()
.get(url + endpoint);
System.out.println(response.jsonPath().prettyPrint());
Assertions.assertEquals(200, response.statusCode());
response = given()
.contentType("application/json")
.body(jsonAsMap)
.when()
.post(url + endpoint);
Assertions.assertEquals(200, response.statusCode());
MatcherAssert.assertThat("application/json", equalTo(response.contentType()));
System.out.println(response.jsonPath().prettyPrint());
}
}
Rest Assured Scala Tutorial
From the REST Assured 2.6.0 version, we can also use this library when writing our tests in Scala. The only change compared to Java is that then() is reserved for keywords in Scala, so Scala support for REST Assured uses the word Then() for assertions.
To start we need:
- Basic https://mvnrepository.com/artifact/io.rest-assured/rest-assured
- Scala support https://mvnrepository.com/artifact/io.rest-assured/scala-support
- JUnit (unit tests, needed here for the assertion)
https://mvnrepository.com/artifact/junit/junit
https://mvnrepository.com/artifact/org.junit.jupiter/junit-jupiter-api - Jackson Core (parser and generator abstractions used by Jackson Data Processor) https://mvnrepository.com/artifact/com.fasterxml.jackson.core/jackson-databind
- Scribe Java (Simple OAuth library for Java or Scala here)
https://mvnrepository.com/artifact/com.github.scribejava/scribejava-httpclient-okhttp
https://mvnrepository.com/artifact/com.github.scribejava/scribejava-apis
Note: For me, a higher version of Scribe Java API’s than 2.5.3 generates an error java.lang.NoClassDefFoundError: com/github/scribejava/core/model/AbstractRequest
Here, I have used the same API as in the previous example. In Scala, the syntax of Rest Assured looks really similar, in only a few lines we have a full POST request, I have checked the status code and content type, so there are two assertions.
Note: It should be pointed out that all of our code in Rest Assured can be written in one line, but for the sake of readability, I have put the code line by line.
import java.util
import io.restassured.RestAssured.given
import io.restassured.module.scala.RestAssuredSupport.AddThenToResponse
import org.junit.{Test}
class RestScala {
private val url = "https://localhost:8080/"
private val endpoint = "items"
@Test def crudTest(): Unit = {
val jsonAsMap = new util.HashMap[String, Any]()
jsonAsMap.put("name", "Scalac")
jsonAsMap.put("price", 100)
val response = given()
.body(jsonAsMap)
.contentType("application/json")
.when()
.post(url+endpoint)
.Then()
.assertThat()
.contentType("application/json")
.and()
.statusCode(200)
}
}
Summary
Rest Assured can be useful when it comes to testing our API, but we do not have to write code only in Java; it is also possible in Scala. With syntax gherkin and minimal coding skills, Rest Assured is not only friendly for code creators but also for the people reading it. I hope you have learned something new and I have managed to interest you in the subject of API testing.
See also